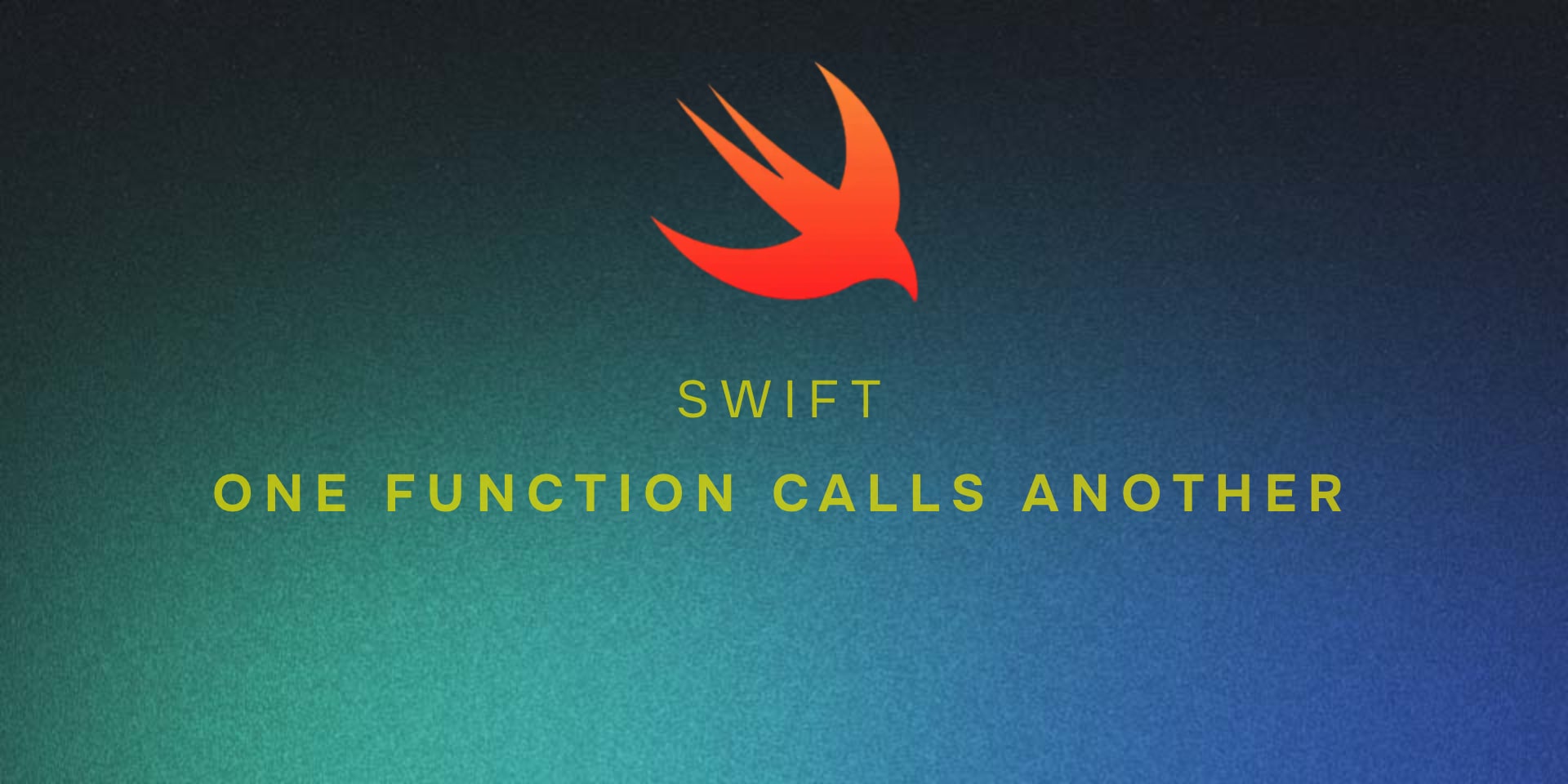
Function Chaining
Function Chaining in Swift: How to Use It to Improve Your Code
Function chaining in Swift is a powerful technique that allows one function to call another, making code organization easier and improving readability. In this article, we’ll explore how this technique can be useful through a practical example adapted to a more realistic context.
Practical Example
Imagine you’re working on an app for a local business in Miami and need to display personalized information for users, such as a greeting and the typical formatting of their phone numbers. The following code demonstrates how functions can be chained to achieve this:
// First function: displays a personalized greeting
func showGreeting(nickname: String) {
print("Hello, \(nickname)!\nThis function is designed to greet users.")
showLocalPhoneNumber(phoneNumber: "123-4567")
}
// Second function: displays the local phone prefix
func showLocalPhoneNumber(phoneNumber: String) {
let prefix: Int = 305
print("If you're from Miami, your typical phone number would be \(prefix)-\(phoneNumber).")
}
// Function that gets a username and calls the previous functions
func getUserName() -> String {
let userName: String = "Luis Fernando"
showGreeting(nickname: "Luis")
return userName
}
// Initial call that starts the function chain
getUserName()
Advantages of Function Chaining
- Clarity and Organization
Function chaining allows you to break code into small, manageable parts. Instead of having a single function that performs multiple tasks, you can have several specialized functions that call each other, making the code easier to understand and maintain.
- Code Reusability
By separating the greeting and phone logic into distinct functions, they can be reused in other parts of the program without duplicating code.
- Maintainability
If you need to change the phone number format or greeting message in the future, you only have to modify a specific function, reducing the risk of errors.
Function chaining is a simple yet effective technique that improves the structure and clarity of Swift code. By dividing your code into specialized functions and logically connecting them, you can create cleaner, more readable, and easier-to-maintain applications.
If you’re learning Swift and want to enhance your skills, try chaining functions in your own projects. This practice will not only help you better organize your code but also allow you to work more efficiently as your projects grow in complexity.